Mastering REST: A Comprehensive Beginner’s Guide
Wed Sep 13 2023
|API Archive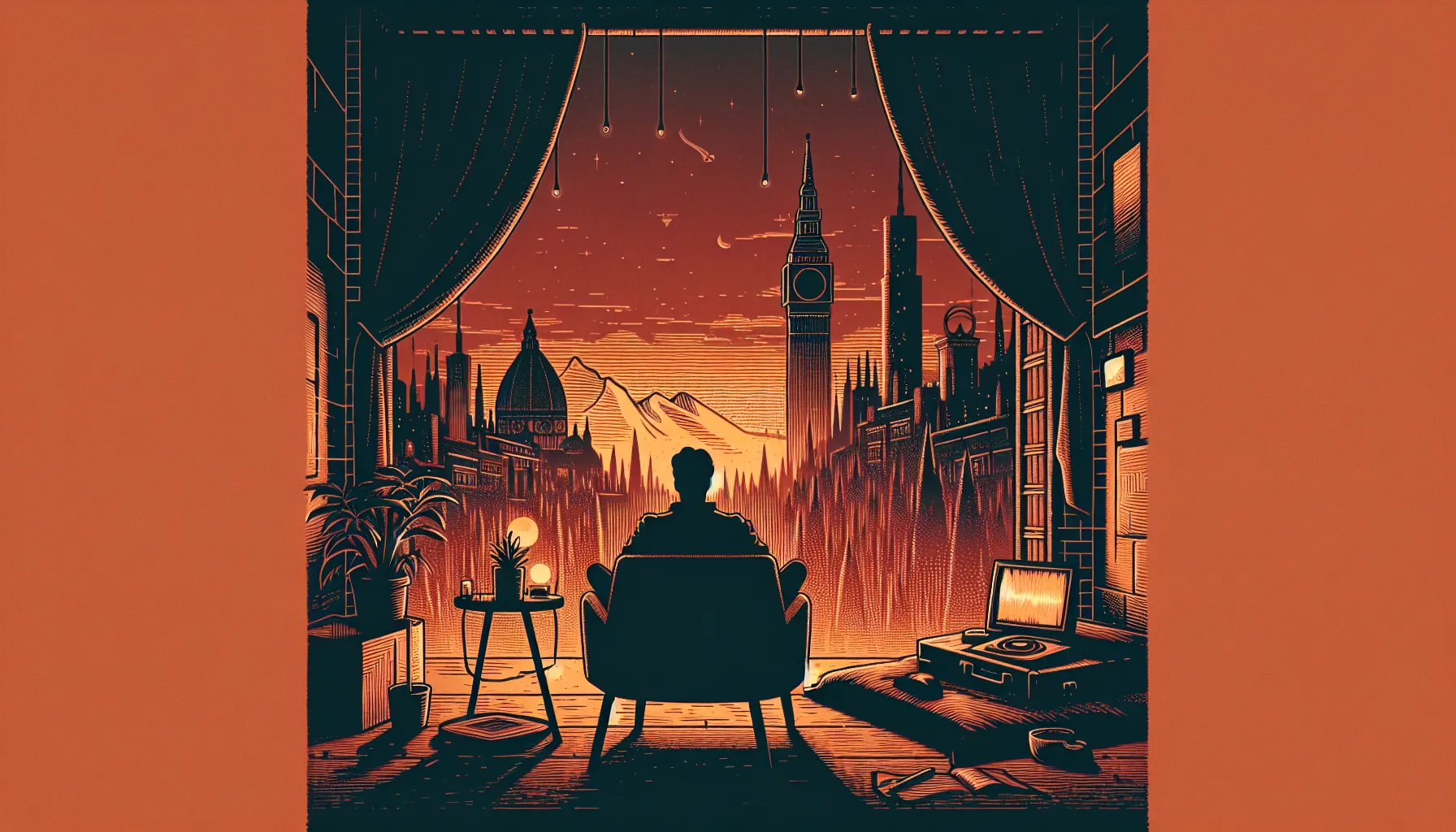
Understanding REST: The Basics
REST (Representational State Transfer) is an architectural style for building distributed, modular systems that communicate over the web. At its core, REST defines a set of constraints and best practices for creating web services that focus on scalability, flexibility, and simplicity.
A RESTful web service exposes resources through URIs that clients can access using standard HTTP methods. Resources are represented in formats like JSON and XML for easy serialization and transport. REST systems are stateless, meaning the server doesn’t need to retain information between requests. This improves scalability and reduces server load.
The benefits of REST include:
- Simplicity – No complex toolkits needed. Use HTTP protocols for requests/responses.
- Flexibility – Data is not tied to resources or methods. Many formats supported.
- Scalability – Stateless server so highly scalable. Caching helps efficiency.
- Portability – Any device can access REST APIs via HTTP.
- Reliability – Can leverage existing HTTP infrastructure, security, etc.
Overall, the REST approach helps integrate systems and data sources through reusable web standards. It is simple yet powerful.
The Evolution of REST and Its Importance
REST originated in a 2000 PhD dissertation by Roy Fielding. He observed that HTTP, the protocol of the World Wide Web, already followed the principles of a generic web-based architecture. This style came to be known as REST, and it has become the standard for building web APIs.
Since 2000, REST has steadily gained popularity as more and more public web APIs adopted it:
- 2005 – First wave with APIs from Amazon, Yahoo, Google, eBay
- 2007 – Growth with APIs from Facebook, Twitter
- 2009 – Explosion with APIs from thousands of technology companies
- Today – REST dominates web APIs and is the standard approach
There are several key reasons why REST grew to prominence:
- Leverages simpler HTTP protocols rather than complex RPC or SOAP
- Improved performance, reliability and scalability
- Better integration across systems and languages
- Higher developer productivity due to easier data access
As the world moves increasingly to distributed apps and web services, REST has become indispensable for modern web development. Its constraints and principles help structure interactions in fast-growing ecosystems. The importance and ubiquity of REST will only continue increasing going forward.
REST vs. SOAP: Key Differences
SOAP (Simple Object Access Protocol) is an XML-based messaging protocol for accessing web services. It preceded REST and achieved early popularity, but has mostly been superseded by REST in modern web development.
Some key differences between the REST and SOAP web services architectures:
Simplicity – SOAP uses complex XML schemas and requires more specialized client/server knowledge. REST uses simpler architecture and HTTP requests/responses.
Caching – REST permits caching data to improve performance. SOAP interactions are stateful so no caching.
Statelessness – REST systems are stateless while SOAP retains state between messages. This impacts scalability.
Flexibility – REST has fewer formatting restrictions so more media types supported. SOAP only works with XML formatting.
Performance – REST interactions are faster and use less bandwidth thanks to simpler messaging and caching.
Portability – REST uses ubiquitous HTTP so available for wider range of platforms. SOAP less portable.
In summary, REST provides faster, more scalable and flexible web interactions compared to SOAP. This has solidified its status as the premier web API architecture over the past decade.
Principles of RESTful Architecture
For an API interface to be considered RESTful, it must conform to the formal REST architectural constraints:
Client-Server Separation
The interface separates client concerns from data storage concerns. This improves portability and scalability.
Statelessness
No client session data or context is stored server-side between requests. Each request has enough info to complete itself.
Cacheability
REST responses indicate if request data can be cached to enhance performance. Caching reduces client-server interactions.
Layered System
REST allows hierarchical system layers where components only interact with the immediate layer. Improves manageability.
Uniform Interface
REST requires use of uniform, predefined operations to manipulate resources through representations. Simplifies architecture.
Code on Demand (Optional)
REST permits code download for extensibility. This simplifies clients by reducing pre-included features.
By leveraging these constraints, RESTful interfaces deliver increased scalability while maintaining flexibility. The constraints also facilitate REST’s focus on reusability and loose coupling between client/server.
Building Your First REST API: A Step-by-Step Guide
Designing and implementing a REST API may sound daunting but can be straight-forward by following these key steps:
1. Define Resources
First identify the key objects that will be exposed as resources. These should be nouns that make natural URIs. For example, for blog content, resources may be posts, comments, categories, users, etc.
2. Determine Operations
Decide the supported operations on each resource – create, read, update, delete. These map to HTTP methods: POST, GET, PUT, DELETE. Not all resources need to allow all operations.
3. Establish URIs
URIs should include the resource name and unique identifier for each resource so it can be located unambiguously. For example:
/posts
/posts/{post_id}
4. Represent Resources
Choose a representation format like JSON or XML for transferring resource state. The format should be highly portable and serializable.
5. Handle Requests Properly
Use HTTP methods and assess request headers/bodies appropriately:
– GET – Read resource state from URI
– PUT – Update state of existing URI resource
– POST – Create new resource from supplied representation
– DELETE – Delete resource specified by URI
6. Return Correct Responses
Respond with appropriate HTTP status codes and headers:
– 200 OK – Success with response body containing representation
– 201 Created – Successfully created new resource
– 204 No Content – Resource deleted successfully
By following these guidelines you can design and build highly effective REST APIs.
Exploring RESTful HTTP Methods
REST leverages standard HTTP protocol methods to perform CRUD (create, read, update, delete) operations on resources. The primary HTTP methods used in REST APIs are:
GET
- Retrieve a resource
- Idempotent – Same result if called once or multiple times
- Usually no request body, just URI
- 200 (OK) expected response indicating success
Example: Retrieving a blog post
GET /posts/123
POST
- Create a new resource
- Not idempotent
- Contains request body with details of resource to create
- 201 (Created) expected response
Example: Creating new blog post
POST /posts
{
"title" : "New Post",
"content" : "This is a new post!"
}
PUT
- Update existing resource
- Idempotent
- Contains request body with updated resource details
- 200 (OK) expected response
Example: Updating existing blog post
PUT /posts/123
{
"title" : "Updated Post Title"
}
DELETE
- Delete a resource by URI
- Idempotent
- No request body needed
- 204 (No Content) expected response
Example: Deleting blog post
DELETE /posts/123
So in summary, HTTP methods provide the fundamental operations for manipulating resources in a RESTful system.
Handling Data and Serialization in REST
A key aspect of REST APIs is handling data serialization and deserialization. Because REST permits many data formats, the client and server need to agree on a mutually supported format. This is achieved through content negotiation.
The main mechanisms for negotiating data formats are:
Accept Header
The client sends an Accept header indicating preferred formats:
Accept: application/json
Format Extension
The client specifies format in the resource URI:
GET /posts/123.json
Query Parameter
Format is specified via query string parameter:
GET /posts/123?format=xml
Once a format is agreed, common options for REST APIs include:
JSON – Wide support across languages. Easy to parse and great for web.
XML – More verbose than JSON but widely used. Supports validation via XML schemas.
YAML – Human-readable so great for configuration data.
Plain Text – Simplest option. Used for things like string or number resources.
REST does not limit data formats. Any widely accepted serializable format is fine. The key is establishing the format via content negotiation.
Security Practices for REST APIs
As REST APIs provide access to key systems and data sources, securing them is extremely important. Some essential practices include:
HTTPS Everywhere – Always use HTTPS TLS encryption for requests/responses. This prevents eavesdropping.
Authentication – Require credentials to verify valid user identity before allowing access. Options like API keys, OAuth, Basic Auth, tokens.
Access Control – Limit which endpoints, HTTP verbs and data each user role can access. This least privilege model limits damage from compromised credentials.
Input Validation – Rigorously validate all input data to prevent attacks like SQL injections or stored XSS.
Audit Trails – Log all access requests and responses to identify potential security issues.
Rate Limiting – Prevent brute force attacks by limiting number of requests permitted per timeframe.
TLS Certificate Pinning – The client rejects server certificate unless signed by specific Certificate Authority to prevent MITM attacks.
There are many other protections depending on the particular system, but these form a fairly comprehensive initial REST API security baseline. They help ensure APIs are safe and performant for consumption by external partners and the public web.
Testing and Debugging RESTful Services
Rigorously testing REST APIs is critical given complex input permutations and reliance from client apps. Key testing principles include:
Unit Testing – Test serialization, URIs, HTTP handling logic etc in isolation. Mock other components.
Integration Testing – Test complete request/response flow including middleware. Use test doubles to simulate databases and networks.
Functional Testing – Test from a client perspective. Use actual front-end apps if possible or simulate with scripts. Focus on business logic.
Security Testing – Actively probe for vulnerabilities like injections, data leaks, rate limit bypasses. Utilize automation tools like scanners.
Load Testing – Simulate expected concurrent users and request volumes. Use production-sized data sets. Check for performance issues.
Regression Testing – Conduct extensive retesting after any code or environment changes to ensure no regressions.
In addition to testing, REST APIs should provide comprehensive debugging information:
- Meaningful status codes, headers and messages
- Detailed request/response logging
- API debugging options for more verbosity
-traces/profiling for investigating performance
Testing and debugging are intricate disciplines but form the foundation for bulletproof, production-grade REST APIs.
Advanced REST Concepts: Beyond the Basics
As mastery over REST develops, there are a number of powerful advanced concepts that can transform API capabilities:
Hypermedia – Having resources supply controls for available transitions helps decouple clients from specific API structure. Clients interact through hypermedia instead of hardcoded paths.
HATEOAS – Acronym meaning Hypermedia As The Engine Of Application State. Making the API navigable via hyperlinks reduces client coupling and keeps business logic on server.
Versioning – Allows new API iterations without breaking existing clients. Typically done via request headers, custom media types or URIs. Enables smooth evolution.
API Descriptions – Machine-readable metadata providing info about API capabilities, parameters, auth etc. Formats like OpenAPI and JSON Schema help autogenerate documentation and accelerate development.
Webhooks – Event notification mechanism where apps subscribe to updates from API resources they are interested in rather than polling. Very effective for real-time apps.
Learning concepts like these will vastly expand the sophistication possible with REST APIs and client apps.
Leveraging REST APIs in Web Development
REST has become the defacto standard for web API development due to its simplicity, flexibility and scalability. Learning it unlocks the capability to integrate powerful services into websites and apps.
For front-end developers, REST skills empower fetching and displaying dynamic data from APIs rather than just static pages. React and other JavaScript frameworks make consuming REST APIs trivial.
For back-end developers, implementing REST APIs allows public reuse of application logic and data while maintaining security controls. Python, Node, Java and other server languages have robust REST frameworks available.
For mobile developers, accessing device-friendly REST APIs enables great flexibility when building iOS and Android apps without needing to design custom backend protocols.
The recent API economy boom has been largely driven by product developers leveraging REST to quickly integrate useful services like payments, messaging, storage, search and more into apps via simple API consumption.
As connectivity and composability increase the importance of web services, having REST knowledge will be even more indispensable for modern web development.
Navigating Common REST Challenges
While very effective, REST does come with a few common pain points to navigate:
Over-Fetching – Requires more roundtrips compared to graph schemas if an entity has many linked relations in data model. Need disciplined approach to stitch together data ahead of time based on expected usage patterns.
Versioning – APIs evolve so smooth versioning strategies are important to avoid breaking existing consumers as changes rolled out. Schema evolution best handled in compatible fashion via guidelines like API lifecycle management.
Documentation – Hard to keep accurate and updated given rapid agile development lifecycles. Autogenerated API description formats emerging to help alleviate manual doc creation and decay.
Security – More exposure vectors with wider API surface area and accessibility, increasing security considerations around authentication, authorization, data validation, rate limiting and more compared to monoliths.
Learning REST certainly has a learning curve but pays dividends in building sophisticated, connected systems. Despite common challenges, REST remains the most simple and elegant approach for delivering enterprise-grade API capabilities.